Spring AI快速入门-基于DeepSeek&智谱实现聊天应用
Spring AI快速入门-基于DeepSeek&智谱实现聊天应用
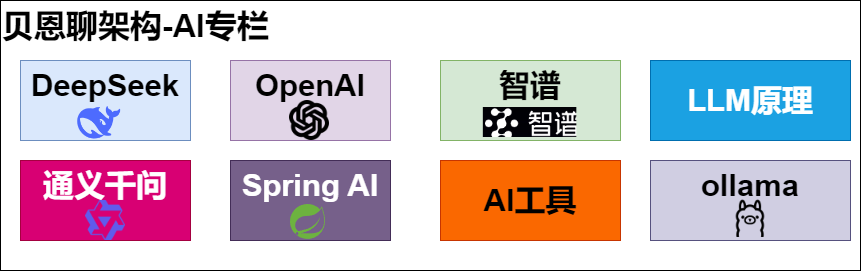
上一篇文章分享了IntelliJ IDEA集成主流 AI 编程助手及特性介绍今天这篇文章我们来分享Spring AI快速入门-SpringAI 基于DeepSeek&智谱实现聊天应用。
文章最后可以加入免费的Java技术栈沟通社群,一起探讨Java/你的产品如何与AI结合,请按照要求加入。在群中可以聊开发、系统设计、架构、行业趋势、AI等等话题
完整代码在文章最后,如果觉得本篇文章对你有用,记得点赞、关注、收藏哦。你的支持是我持续更新的动力!
AI专栏软件环境
- IntelliJ IDEA2024.3.2.2
- Spring AI 1.0.0-SNAPSHOT
- Spring Boot 3.4.2
- Spring 6.2.2
- 智谱AI大模型
- DeepSeek
- JDK 17.0.12
我们先看本篇文章对应的项目结构,请看下图
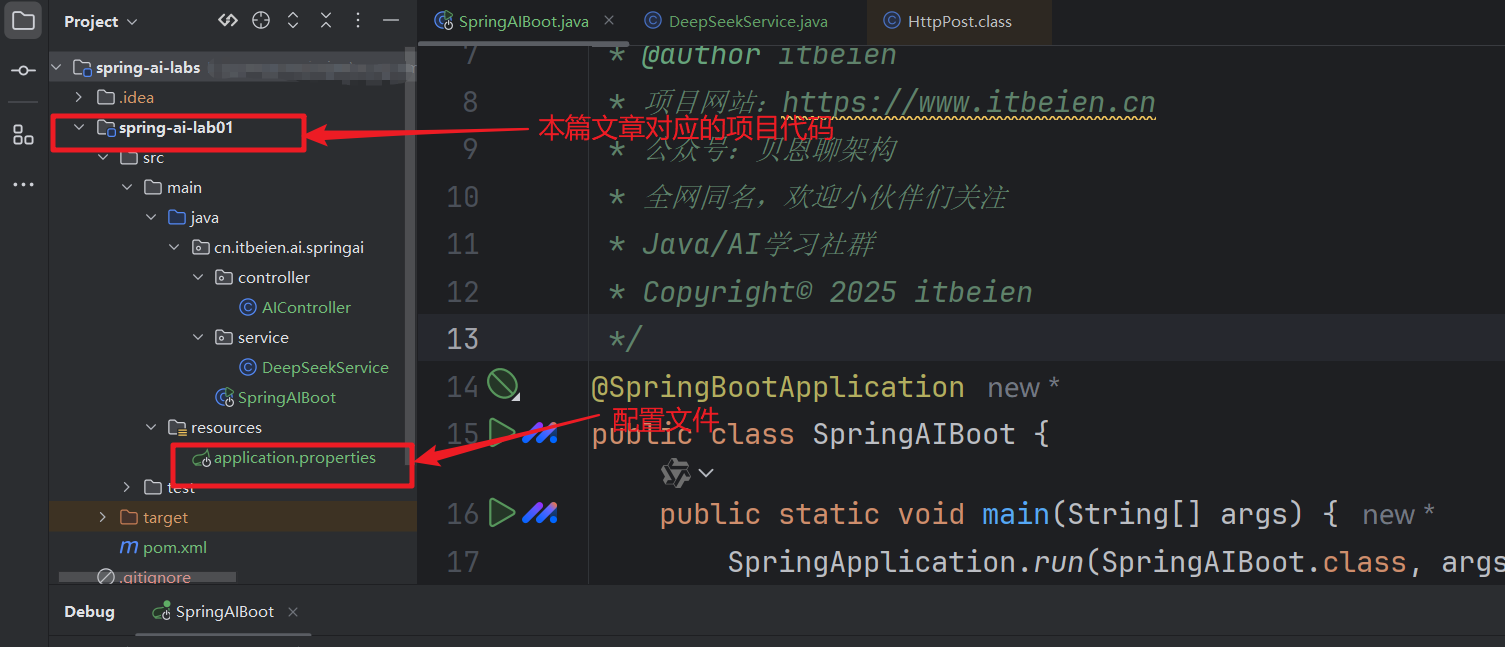
1 Spring AI
Spring AI是一个用于AI项目的应用框架。它的目标是将Spring生态系统设计原则(如可移植性和模块化设计)应用于AI领域,并促进像开发Spring企业应用一样构建AI领域应用程序。
Spring AI能做什么?
- 支持目前主流大语言模型平台,例如 OpenAI、Microsoft、Amazon、Google、智普 和 Huggingface
- 支持阻塞与流式的文本对话
- 支持图像生成(当前仅限OpenAI的dall-e-*模型和SD)
- 支持嵌入模型
- 支持LLM生成的内容转为POJO
- 支持主流的向量数据库或平台:Azure Vector Search, Chroma, Milvus, Neo4j, PostgreSQL/PGVector, PineCone, Qdrant, Redis 和 Weaviate
- 支持函数调用
- 支持自动装配和启动器(与Spring Boot完美集成)
- 提供用于数据处理工程的ETL框架
GitHub:https://github.com/spring-projects/spring-ai
官方文档:https://spring.io/projects/spring-ai
智谱AI大模型开放平台:https://bigmodel.cn/
deepseek开放平台:https://platform.deepseek.com/
2 项目搭建
2.1 父工程pom依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.itbeien.ai</groupId>
<artifactId>spring-ai-labs</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>pom</packaging>
<modules>
<module>spring-ai-lab01</module>
</modules>
<properties>
<maven.compiler.source>17</maven.compiler.source>
<maven.compiler.target>17</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<springai.version>1.0.0-SNAPSHOT</springai.version>
<spring-boot-dependencies-version>3.4.2</spring-boot-dependencies-version>
<lombok.version>1.18.32</lombok.version>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>${spring-boot-dependencies-version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bom</artifactId>
<version>${springai.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>${lombok.version}</version>
</dependency>
</dependencies>
</dependencyManagement>
</project>
2.2 子工程pom依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>cn.itbeien.ai</groupId>
<artifactId>spring-ai-labs</artifactId>
<version>1.0-SNAPSHOT</version>
</parent>
<artifactId>spring-ai-lab01</artifactId>
<dependencies>
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-zhipuai-spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.14</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
</project>
2.3 配置信息
spring.ai.zhipuai.api-key=你的api-key
spring.ai.deepseek.api-key=你的api-key
spring.ai.deepseek.api-url=api-url
2.4 controller
package cn.itbeien.ai.springai.controller;
import cn.itbeien.ai.springai.service.DeepSeekService;
import jakarta.annotation.Resource;
import lombok.extern.slf4j.Slf4j;
import org.springframework.ai.chat.client.ChatClient;
import org.springframework.ai.zhipuai.ZhiPuAiImageModel;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
/**
* @author itbeien
* 项目网站:https://www.itbeien.cn
* 公众号:贝恩聊架构
* 全网同名,欢迎小伙伴们关注
* Java/AI学习社群
* Copyright© 2025 itbeien
*/
@RestController
@RequestMapping("/ai")
@Slf4j
public class AIController {
private final ChatClient chatClient;
@Autowired
private DeepSeekService deepSeekService;
public AIController(ChatClient.Builder chatClientBuilder) {
this.chatClient = chatClientBuilder.build();
}
@GetMapping("/hello")
String generation(@RequestParam("userInput") String userInput) {
return this.chatClient.prompt()
.user(userInput)
.call()
.content();
}
@GetMapping("/deepseek")
String deepseek(@RequestParam("userInput") String userInput) {
try {
return deepSeekService.askDeepSeek(userInput);
}catch (Exception e){
log.error("调用DeepSeek失败", e);
return "error";
}
}
}
2.5 service
package cn.itbeien.ai.springai.service;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import javax.validation.Valid;
import java.io.IOException;
/**
* @author itbeien
* 项目网站:https://www.itbeien.cn
* 公众号:贝恩聊架构
* 全网同名,欢迎小伙伴们关注
* Java/AI学习社群
* Copyright© 2025 itbeien
*/
@Service
@Slf4j
public class DeepSeekService {
@Value("${spring.ai.deepseek.api-url}")
private String API_URL;
@Value("${spring.ai.deepseek.api-key}")
private String API_KEY;
public String askDeepSeek(String question) throws IOException {
CloseableHttpClient client = HttpClients.createDefault();
// 创建 HTTP POST 请求
HttpPost request = new HttpPost(API_URL);
request.setHeader("Authorization", "Bearer " + API_KEY);
request.setHeader("Content-Type", "application/json; charset=UTF-8");
// 动态构建请求体
String requestBody = String.format(
"{\"model\": \"deepseek-chat\", \"messages\": [{\"role\": \"user\", \"content\": \"%s\"}], \"stream\": false}",
question
);
log.info(requestBody);
request.setEntity(new StringEntity(requestBody,"UTF-8"));
// 发送请求并获取响应
try (CloseableHttpResponse response = client.execute(request)) {
// 返回响应内容
return EntityUtils.toString(response.getEntity());
}
}
}
3 代码测试
3.1 启动项目

3.2 使用apifox进行测试
使用apifox访问ai应用程序,输入http://localhost:8080/ai/hello或http://localhost:8080/ai/deepseek,输入对应聊天参数如下图
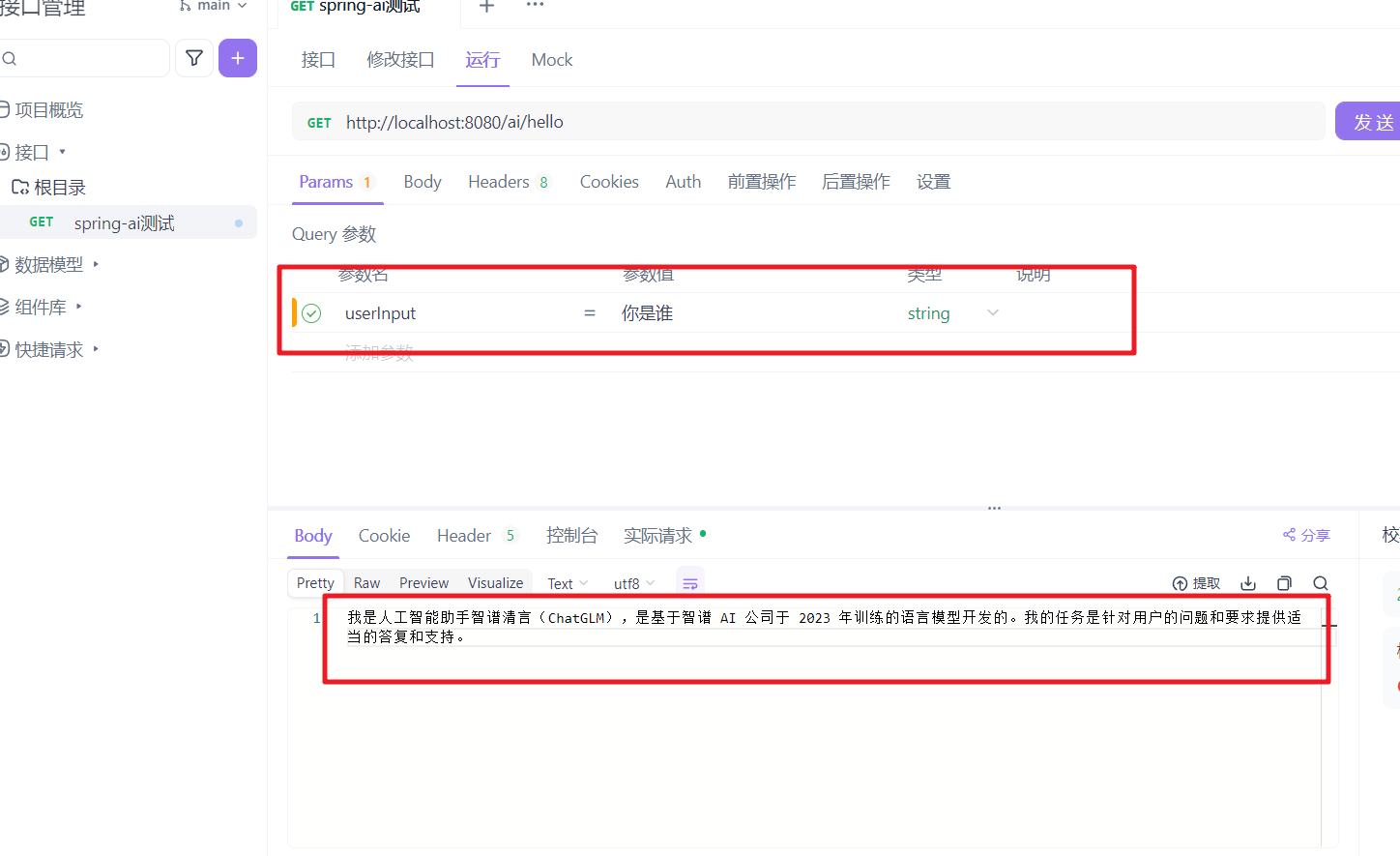
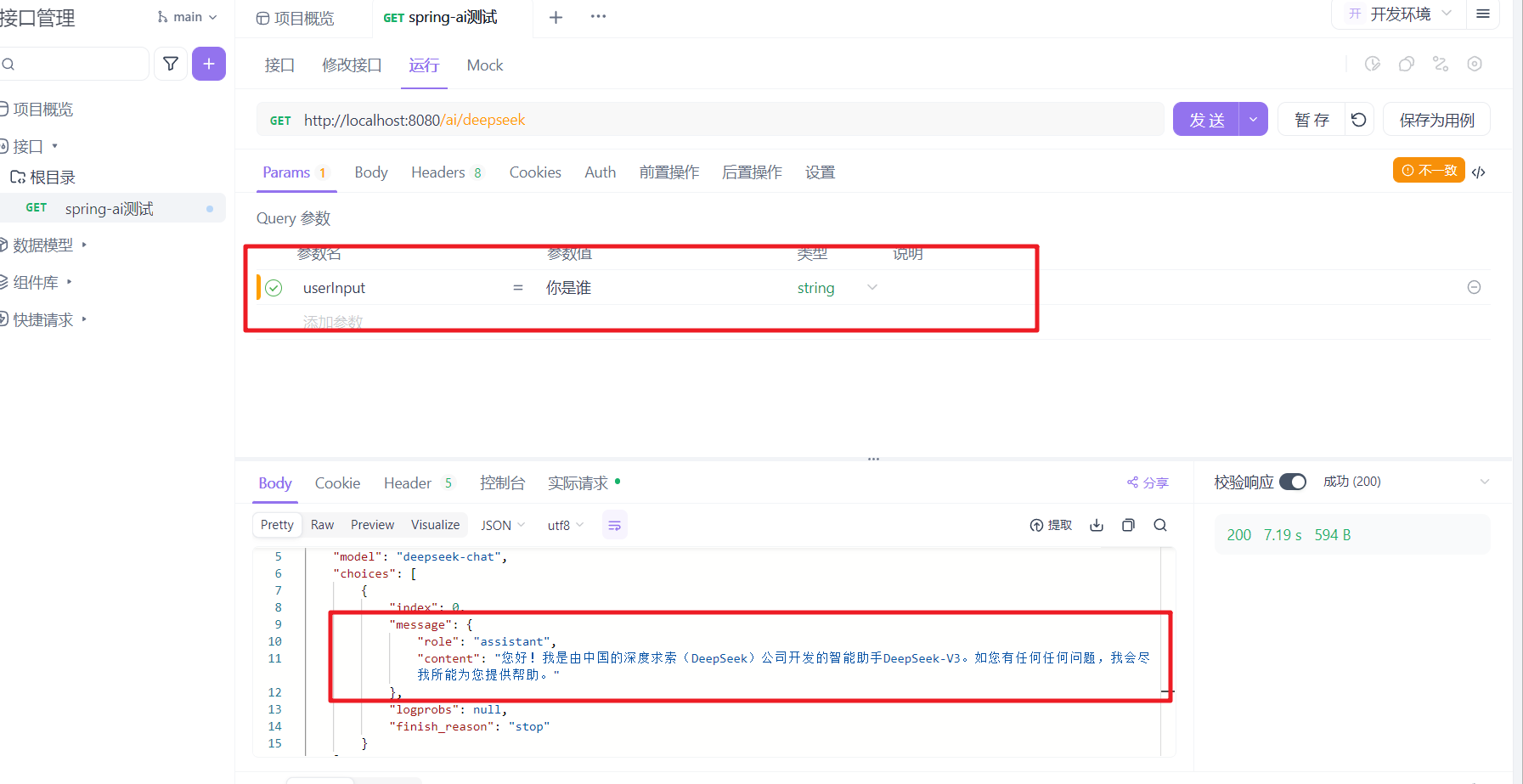
以上就是今天Spring AI快速入门-SpringAI 基于DeepSeek&智谱实现聊天应用全部内容,文章最后有源码下载地址
欢迎大家关注我的项目实战内容itbeien.cn,一起学习一起进步,在项目和业务中理解各种技术。
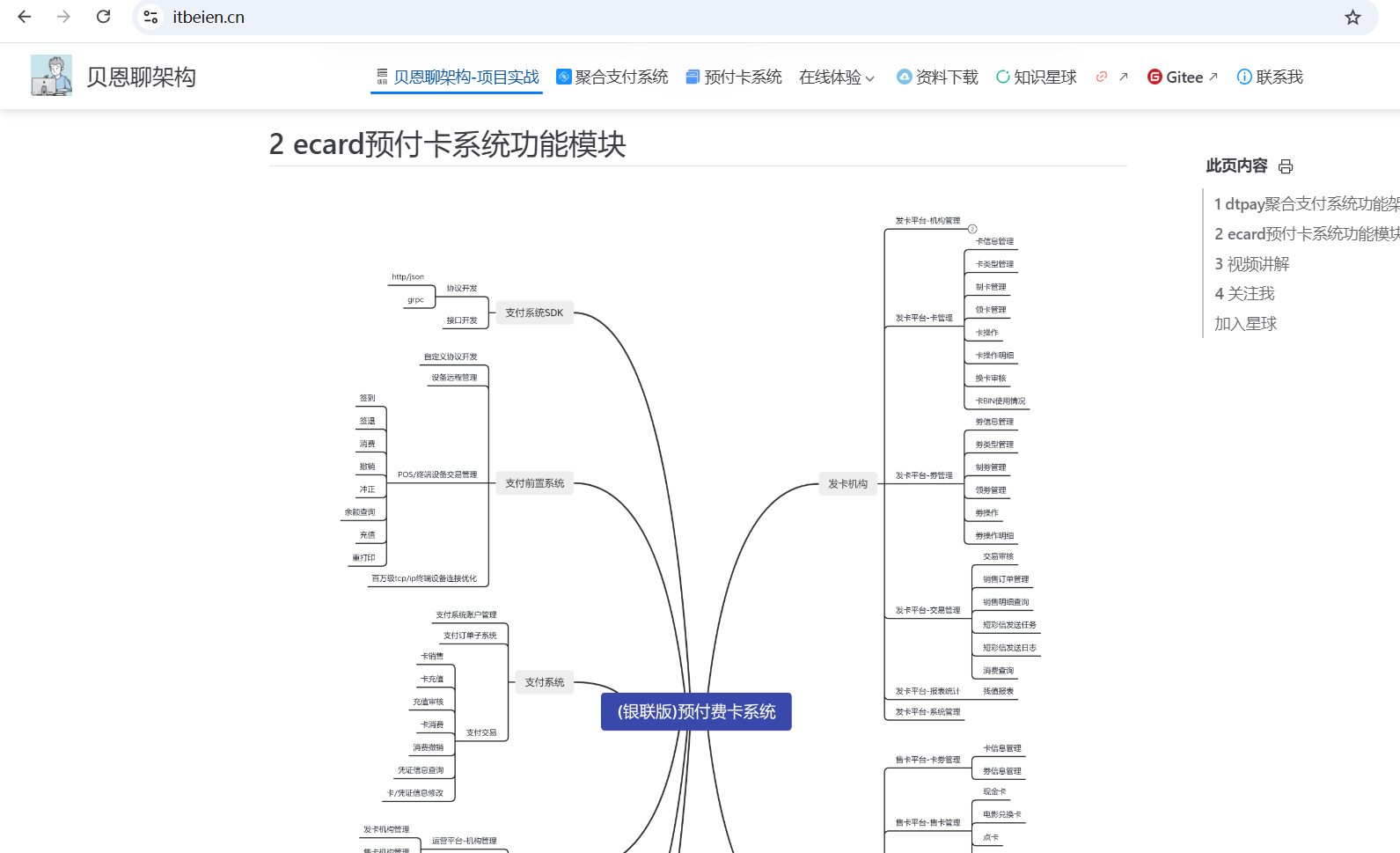
欢迎沟通交流Java/AI技术和支付业务,一起探讨大模型应用/SAAS多租户/聚合支付/预付卡系统业务、技术、系统架构、微服务、容器化。并结合大模型应用/SAAS多租户/聚合支付系统深入技术框架/微服务原理及分布式事务原理。加入我的知识星球吧
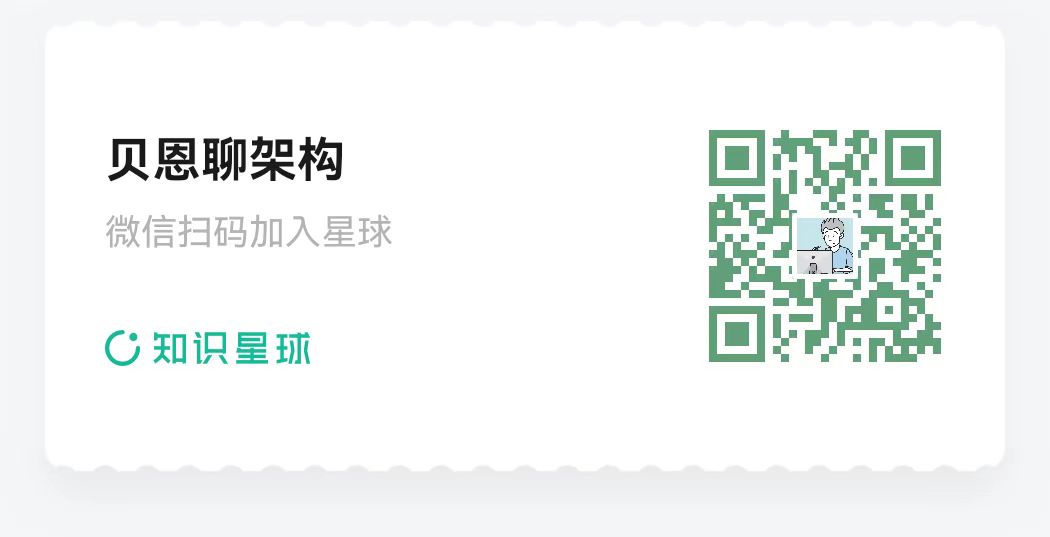
AI专栏
01IDEA&VsCode集成DeepSeek-V3 API提高编程效率
02IntelliJ IDEA集成主流 AI 编程助手及特性介绍
SpringBoot3专栏
01SpringBoot3专栏-SpringBoot3.4.0整合Mybatis-plus和Mybatis
02SpringBoot3.4.0结合Mybatis-plus实现动态数据源
03mapstruct对象映射在Springboot3中这样用就对了
04RocketMQ5.3.1集成SpringBoot3.4.0就这样简单
05SpringBoot3.4.0整合Redisson实现分布式锁
06MySQL增量数据同步利器Canal1.1.7环境搭建流程
07SpringBoot3.4.0集成Canal1.1.7实现MySQL实时同步数据到Redis
08基于Docker-SpringBoot3.4.0集成Apache Pulsar4.0.1实现消息发布和订阅
09SpringBoot3.4.0整合消息中间件Kafka和RabbitMQ
10SpringBoot3.4.0整合ActiveMQ6.1.4
11SpringBoot3整合Spring Security6.4.2 安全认证框架实现简单身份认证
12SpringBoot3.4.1和Spring Security6.4.2实现基于内存和MySQL的用户认证
13SpringBoot3.4.1和Spring Security6.4.2结合OAuth2实现GitHub授权登录
14SpringBoot3.4.1和Spring Security6.4.2结合JWT实现用户登录
16SpringBoot3.4.1基于MySQL8和Quartz实现定时任务管理
跟着我学微服务系列
01跟着我学微服务,什么是微服务?微服务有哪些主流解决方案?
05SpringCloudAlibaba之图文搞懂微服务核心组件在企业级支付系统中的应用
06JDK17+SpringBoot3.4.0+Netty4.1.115搭建企业级支付系统POS网关
07JDK17+SpringCloud2023.0.3搭建企业级支付系统-预付卡支付交易微服务
08JDK17+Dubbo3.3.2搭建企业级支付系统-预付卡支付交易微服务
09JDK17+SpringBoot3.3.6+Netty4.1.115实现企业级支付系统POS网关签到功能
贝恩聊架构-项目实战地址
欢迎大家一起讨论学习,加我备注"JAVA"拉你进入技术讨论群,在技术学习、成长、工作的路上不迷路!加我后不要急,每天下午6点左右通过!营销号免入
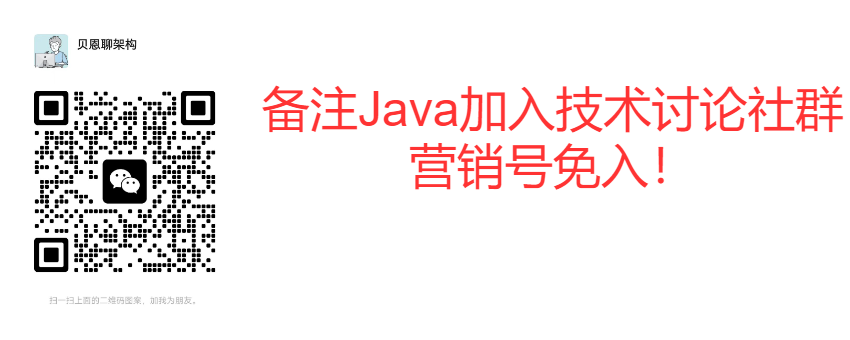
4 源码地址
贝恩聊架构-SpringBoot3专栏系列文章、资料和源代码会同步到以下地址,代码和资料每周都会同步更新
该仓库地址主要用于贝恩聊架构-SpringBoot3专栏、基于企业级支付系统,学习微服务整体技术栈
